Vectors in C++
Introduction
An std::vector object acts like an array but with a dynamic size, meaning that it can grow or shrink as the program executes. This in is contrast to static size data structures which must have their sizes determined at compile time. The elements of a vector are represented contiguously in memory, meaning that elements of the vector are stored next to each other, so iterating over a pointer to the vector will have the effect of looping over the elements of the vector.
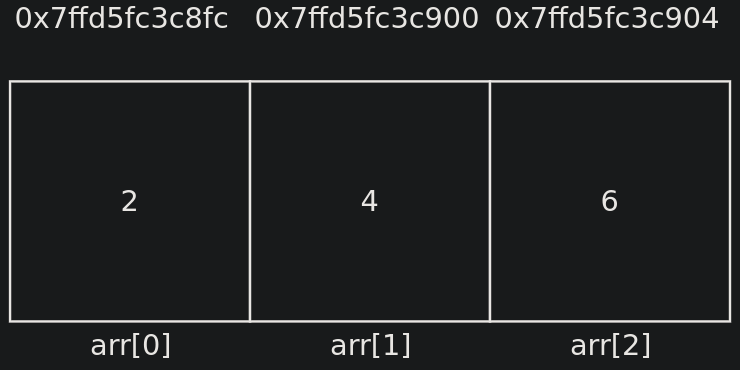
Usage
Below is an example of an std::vector in use. The vector has an initial size and capacity of 3, but the size of the vector increases as more elements are added.
std::vector<int> vec = {2, 4, 6};
std::cout << "Vector size after: " << vec.size() << '\n';
std::cout << "Vector capacity after: " << vec.capacity() << '\n';
vec.push_back(8);
vec.push_back(10);
for (int i = 0; i < vec.size(); i++) {
std::cout << "At index " << i << ": " << vec.at(i) << '\n';
}
std::cout << "Vector size after: " << vec.size() << '\n';
std::cout << "Vector capacity after: " << vec.capacity() << '\n';
std::cout.flush();
Resulting in the output:
Vector size before: 3
Vector capacity before: 3
At index 0: 2
At index 1: 4
At index 2: 6
At index 3: 8
At index 4: 10
Vector size after: 5
Vector capacity after: 6
The method push_back(T)
adds a new element to the end of the vector, but if doing so would exceed the capacity of the vector, the vector is resized such that it has enough space to store not only this new element, but several. This is because the operation of resizing a vector is of linear time complexity, so by doing this the number of resize operations are reduced.
The reason for this time complexity is that when the vector is resized, the underlying elements of the array need to be moved to a new data allocation. However, if there is enough space for the element to be added, then the time complexity of adding it to the vector is O(1).
The array can also be manually resized using 3 methods: resize(int)
, reserve(int)
, and shrink_to_fit()
methods. resize(int)
will set the capacity of the vector to the numeric value passed to it, whereas reserve(int)
will increase the capacity of the vector by the provided value. Note that if a value is passed to resize(int) that is lower than the current capacity of the vector, its capacity remains the same but its size decreases. The shrink_to_fit()
method will reduce the capacity of the array so that it matches its size.